clair chin one line
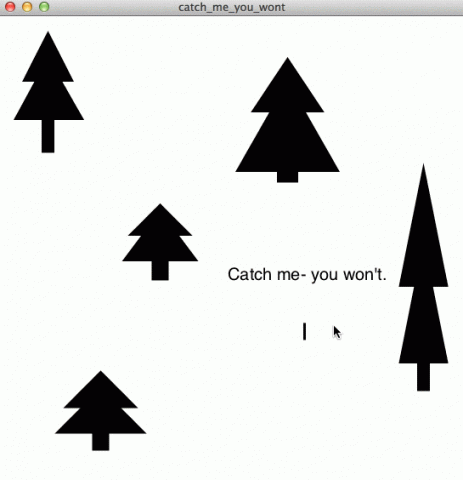
PFont f;
int oneHidingSpotX = 58;
int oneHidingSpotY = 93;
int twoHidingSpotX = 339;
int twoHidingSpotY=142;
int threeHidingSpotX=500;
int threeHidingSpotY=276;
int fourHidingSpotX=190;
int fourHidingSpotY=269;
int fiveHidingSpotX=121;
int fiveHidingSpotY=464;
int lineX=360;
int lineY=360;
int currentTree;
float hidingSpotX=0;
float hidingSpotY=0;
float speed=3;
void setup (){
size(550,550);
smooth();
background(255);
f= createFont(“Helvetica”,20,true);
}
void draw(){
background(255);
///i like trees
///trees from left to right top to bottom
int treeRadius=70;
fill(0);
///tree 1
triangle(58, 20, 30, 75, 86, 75);
triangle(58,50,20,120,98,120);
strokeWeight(15);
strokeCap(SQUARE);
line(58,120,58,160);
///tree 2
strokeWeight(25);
triangle(340,70,320,100,360,100);
triangle(340,100,300,170,380,170);
line(340,160,340,195);
///tree 3
strokeWeight(15);
triangle(500,210,480,310,520,310);
triangle(500,300,480,400,520,400);
line(500,400,500,440);
///tree 4
triangle(190,230,170,250,210,250);
triangle(190,240,160,280,220,280);
strokeWeight(20);
line(190,270,190,310);
///tree 5
triangle(120,430,100,450,140,450);
triangle(120,450,90,480,150,480);
line(120,480,120,510);
//line
strokeWeight(3);
textFont(f);
fill(0);
text(“Catch me- you won’t.”,270,310);
line(lineX,lineY,lineX,lineY+20);
if (distance(mouseX,mouseY,lineX,lineY)<15 && currentTree==0)
{
int tree = (int)random(1,6);
while (currentTree == tree)
{
tree = (int)random(1,6);
}
currentTree = tree;
if (tree==1)
{
hidingSpotX=oneHidingSpotX;
hidingSpotY=oneHidingSpotY;
}
else if (tree==2)
{
hidingSpotX=twoHidingSpotX;
hidingSpotY=twoHidingSpotY;
}
else if (tree==3)
{
hidingSpotX=threeHidingSpotX;
hidingSpotY=threeHidingSpotY;
}
else if (tree==4)
{
hidingSpotX=fourHidingSpotX;
hidingSpotY=fourHidingSpotY;
}
else
{
hidingSpotX=fiveHidingSpotX;
hidingSpotY=fiveHidingSpotY;
}
}
else if (currentTree>0)
{
if (lineX<hidingSpotX)
{
lineX+=speed;
}
else if (lineX>hidingSpotX)
{
lineX-=speed;
}
if (lineY<hidingSpotY)
{
lineY+=speed;
}
else if (lineY>hidingSpotY)
{
lineY-=speed;
}
if (distance(hidingSpotX,hidingSpotY,lineX,lineY)<(1.5*speed))
{
currentTree=0;
}
}
}
float distance (float x,float y,float x1, float y1)
{
float xs=pow(x1-x,2);
float ys=pow(y1-y,2);
float d = pow(xs+ys,0.5);
return d;
}